013 화살표 함수 사용하기
화살표 함수
- function 대신 =>을 사용하며 retrun 문자열을 생략할 수 있음
- ES6에서 등장함
- 콜백 함수에서 this를 bind 해야 하는 문제도 발생하지 않음
- 콜백함수 : 다른 코드의 인수로서 넘겨주는 실행 가능한 코드
- this : 자신이 속한 객체 또는 자신이 생성할 인스턴스를 가리키는 자기 참조 식별자
- this는 함수가 호출되는 방식에 따라 동적으로 결정됨
- this 바인딩 : this를 고정시키는 것
- 참고 문서
import React, { Component } from "react";
class R013_ArrowFunction extends Component {
constructor(props){
super(props);
this.state = {
arrowFunc: 'React200',
num: 3,
};
}
componentDidMount() {
Function1(1);
this.Function2(1,1);
this.Function3(1,3);
this.Function4();
this.Function5(0,2,3);
// Es5 Function
function Function1(num1){
return console.log(`${num1}. Es5 Function`) // 1. Es5 Function
}
}
// Arrow Function
Function2 = (num1, num2) => {
let num3 = num1 + num2; // 2
console.log(`${num3}. Arrow Function: ${this.state.arrowFunc}`)
// 화살표 함수는 this로 컴포넌트의 state 변수 접근 가능
}
// Es5 Function
Function3() {
var this_bind = this;
// 콜백 함수 내부에서는 컴포넌트 this로 접근 불가능
// 콜백 함수 내부에서 this는 window 객체임
// 접근할 수 있는 변수에 this를 백업함 (this_bind)
setTimeout(function() {
console.log(`${this_bind.state.num}. Es5 Callback Function noBind : ${this_bind.state.arrowFunc}`);
},100);
}
// Es5 Function
Function4() {
// 콜백 함수에 함수 밖의 this를 bind해주면, this를 컴포넌트로 사용 가능
setTimeout(function(){
console.log(`4. Es5 Callback Function Bind : ${this.state.arrowFunc}`)
}.bind(this),100)
}
Function5 = (num1, num2, num3) => {
const num4 = num1 + num2 + num3;
// Arrow Function은 this를 bind하지 않아도 됨
setTimeout(() => {
console.log(`${num4}. Arrow Callback Function: ${this.state.arrowFunc}`);
},100);
}
render() {
return(
<h2>[THIS IS ArrowFunction]</h2>
)
}
}
export default R013_ArrowFunction
014 forEach() 함수 사용하기
배열 함수 forEach()
- 배열의 처음부터 마지막 순번까지 모두 작업하는 경우에 간편하게 사용됨
- forEach()는 순번과 배열의 크기 정보를 사용하지 않음
- 0부터 배열의 크기만큼 반복하며 순서대로 배열 값 반환
- 반복문이 실행될 때마다 콜백함수로 결괏값(result)을 받아 새로운 배열(ForEach_newArr)에 넣음
var ForEach_Arr = [3, 3, 9, 8];
var ForEach_newArr = [];
ForEach_Arr.forEach((result) => {
ForEach_newArr.push(result);
})
console.log(`2. ForEach_newArr: [${ForEach_newArr}]`) // [3, 3, 9, 8]
015 map() 함수 사용하기
- 배열 함수 map() 또한 순번과 배열의 크기 변수를 사용하지 않음
- forEach()와 달리 return을 사용해 반환 값을 받을 수 있음
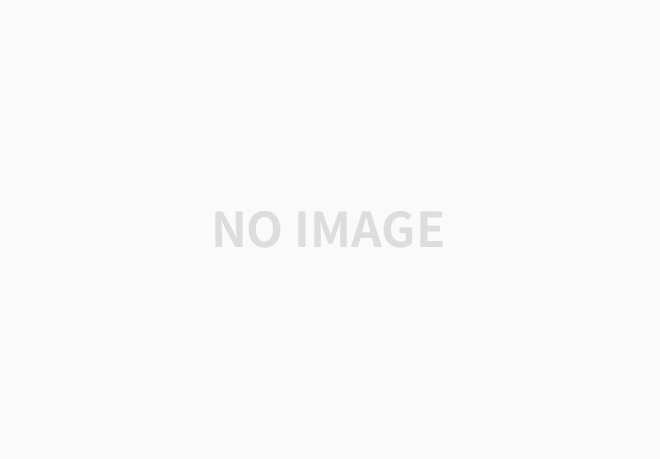